nodejs-backend-architecture-typescript: Building Scalable and Modular Backend Architecture in TypeScript
A brief introduction to the project:
The nodejs-backend-architecture-typescript project is a GitHub repository that provides a comprehensive and well-structured template for building scalable and modular backend applications using TypeScript. It offers a set of best practices and design patterns to develop maintainable and efficient server-side applications. This project is highly relevant for developers who work on Node.js applications, as it helps them streamline their development process and build robust backend architectures.
Project Overview:
The main goal of the nodejs-backend-architecture-typescript project is to provide a solid foundation for building reliable and scalable backend applications. It addresses the common challenges faced by developers, such as code organization, scalability, modularization, and maintainability. By following the guidelines and structure provided by this project, developers can focus on writing business logic and reduce the time and effort spent on architectural decisions.
This project is extremely valuable for developers who are starting a new backend project or want to revamp the architecture of an existing project. It helps them set up a solid foundation with a predefined structure and guidelines, reducing the chances of making common architectural mistakes. The project also emphasizes the use of TypeScript, which adds static typing and improves developer productivity and code quality.
Project Features:
The nodejs-backend-architecture-typescript project offers several key features that contribute to building scalable and maintainable backend applications. Some of these features include:
- Modular Structure: The project follows a modular structure that separates different components of the application, such as controllers, services, models, and routes. This allows for better organization of code and easier maintenance.
- Dependency Injection: The project leverages the Inversion of Control (IoC) pattern and uses dependency injection to manage dependencies between different components. This makes it easier to write testable and reusable code.
- Express.js Integration: The project integrates with the popular Express.js framework, providing a solid foundation for building RESTful APIs or web applications. It includes pre-defined routes, middleware, and error handling logic.
- Authentication and Authorization: The project includes a pre-defined authentication and authorization system using JWT (JSON Web Tokens). This allows developers to add secure user authentication and access control to their applications without having to write the code from scratch.
- Database Integration: The project integrates with popular databases like MongoDB and PostgreSQL, providing a convenient way to perform CRUD (Create, Read, Update, Delete) operations with the database. It also includes database query optimization techniques to improve performance.
Technology Stack:
The nodejs-backend-architecture-typescript project utilizes a variety of technologies and programming languages to build scalable and modular backend architecture. The main technologies used in this project include:
- Node.js: The project is built on top of Node.js, an open-source, event-driven JavaScript runtime. Node.js allows for efficient and scalable backend development.
- TypeScript: TypeScript is a statically typed superset of JavaScript that adds type annotations and other advanced features. It improves code quality, enhances developer productivity, and helps catch errors at compile-time.
- Express.js: Express.js is a popular web application framework for Node.js. It provides a simple and flexible way to build APIs and web applications.
- MongoDB/PostgreSQL: The project supports both MongoDB and PostgreSQL as the backend database. Developers can choose the database that best suits their requirements.
- Mongoose/TypeORM: The project uses Mongoose (for MongoDB) and TypeORM (for PostgreSQL) as Object-Relational Mapping (ORM) libraries. These libraries simplify database operations and provide convenient abstractions for working with the database.
- Jest: Jest is a JavaScript testing framework used in the project for writing unit tests and integration tests. It enables developers to perform automated testing and maintain code quality.
Project Structure and Architecture:
The nodejs-backend-architecture-typescript project follows a well-defined structure and architecture designed to promote modularity, scalability, and maintainability. The project structure is organized as follows:
- `config` directory: Contains configuration files for database connections, environment variables, and other global settings.
- `src` directory: Contains all the application source code. It is further divided into several directories:
- `controllers`: Contains the logic for handling HTTP requests and responses.
- `services`: Contains the business logic and acts as an interface between controllers and models.
- `models`: Contains the database models or schemas used for interacting with the database.
- `routes`: Defines the routes and their corresponding controllers.
- `middlewares`: Contains middleware functions for handling authentication, error handling, etc.
- `tests` directory: Contains all the tests written for the application using Jest framework.
- `README.md` file: Provides detailed instructions on how to set up the project and start using it.
The project architecture follows the MVC (Model-View-Controller) pattern, separating concerns and providing a clear structure for the backend application. It also follows best practices like dependency injection and IoC, resulting in more maintainable and testable code.
Contribution Guidelines:
The nodejs-backend-architecture-typescript project actively encourages contributions from the open-source community. Developers can contribute to this project by submitting bug reports, feature requests, or code contributions through GitHub pull requests.
To contribute to this project, developers are required to follow the guidelines specified in the `CONTRIBUTING.md` file in the project repository. These guidelines outline the process for submitting contributions, coding standards to follow, and documentation requirements. By adhering to these guidelines, developers can ensure that their contributions are well-received and integrated into the project smoothly.
In conclusion, the nodejs-backend-architecture-typescript project provides a robust and scalable template for building backend applications using TypeScript. It offers a set of best practices, design patterns, and features that significantly improve the development process. By following this project's guidelines, developers can build high-quality and maintainable backend architectures, ultimately saving time and effort.
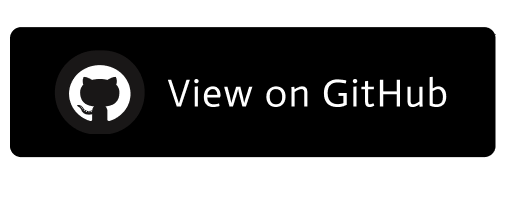