TheAlgorithms/JavaScript: An Extensive Library of Algorithms and Data Structures Implemented in JavaScript
A brief introduction to the project:
TheAlgorithms/JavaScript is a public GitHub repository that provides an extensive library of algorithms and data structures implemented in JavaScript. The project aims to offer a collection of well-tested and documented algorithms that developers can use as a reference or directly incorporate into their own projects. This open-source project encourages collaboration and contribution from the community, making it a valuable resource for developers looking to optimize their code and solve complex problems efficiently.
Project Overview:
TheAlgorithms/JavaScript project serves multiple purposes in the programming community. Firstly, it provides a comprehensive set of algorithms and data structures that can be easily accessed and utilized by developers. These algorithms cover a wide range of problem domains, such as searching, sorting, graph theory, dynamic programming, and much more. By offering a centralized repository of algorithm implementations, the project saves developers significant time and effort, allowing them to focus on building their applications instead of reinventing the wheel.
Additionally, the project also serves as a learning resource for developers who want to enhance their understanding of algorithms and data structures. Each algorithm implementation in the repository is accompanied by detailed explanations, including time and space complexity analysis, code walkthroughs, and examples. This educational aspect of the project makes it a valuable tool for self-study or as a supplement to academic courses.
TheAlgorithms/JavaScript project is relevant and significant in today's software development landscape as it addresses the need for efficient and optimized algorithms. With the increasing complexity of modern applications, having access to well-documented and battle-tested algorithms becomes crucial. By offering a comprehensive collection of algorithms and data structures, the project empowers developers to write high-performance code and tackle complex problem-solving challenges.
Project Features:
The key features of TheAlgorithms/JavaScript project include:
a. Wide Range of Algorithms: The project offers a vast selection of algorithms covering various areas such as searching, sorting, graph theory, dynamic programming, cryptography, and more. This extensive range of algorithms ensures that developers have access to solutions for a wide array of problem domains.
b. Detailed Explanations: Each algorithm implementation in the repository comes with detailed explanations. These explanations cover the algorithm's purpose, approach, time and space complexity analysis, and step-by-step code walkthroughs. These explanations help developers understand the inner workings of the algorithms and how to utilize them effectively.
c. Efficient Implementations: The algorithms in the repository are implemented with a focus on efficiency and performance. They are designed to minimize time and space complexity while maintaining correctness. This emphasis on efficiency ensures that developers can rely on the algorithms for real-world applications without sacrificing performance.
d. Testing and Documentation: The project follows rigorous testing practices, ensuring the correctness and reliability of the algorithm implementations. Additionally, the algorithms are thoroughly documented, providing comprehensive guidance on usage, inputs, outputs, and potential edge cases. These testing and documentation standards ensure that the algorithms can be easily adopted and integrated into any project.
Technology Stack:
TheAlgorithms/JavaScript project utilizes the JavaScript programming language, which is widely used for web development and offers excellent support for high-level programming constructs. JavaScript was chosen as the language of choice due to its popularity, versatility, and widespread adoption in the development community.
In addition to JavaScript, the project also utilizes various libraries and tools for testing, documentation, and code organization. Some notable ones include:
a. Jest: Jest is a JavaScript testing framework used for writing unit tests. It provides a simple and intuitive syntax for writing test cases and offers powerful features such as automatic mocking and code coverage analysis. Jest ensures the reliability and correctness of the algorithm implementations.
b. JSDoc: JSDoc is a documentation generation tool specifically designed for JavaScript projects. It allows developers to add annotations to their source code, which are then parsed to generate comprehensive documentation. JSDoc ensures that the algorithms in the repository are well-documented and easily understandable.
c. GitHub: The project leverages the features and collaborative nature of GitHub to encourage community contributions. Developers can easily submit bug reports, feature requests, and code contributions through pull requests. GitHub also provides version control, issue tracking, and project management capabilities to streamline the development process.
Project Structure and Architecture:
TheAlgorithms/JavaScript project follows a modular structure to organize the different algorithms and data structures. The repository is divided into folders, each corresponding to a specific category or problem domain. For example, there are folders for searching, sorting, graph theory, and more. Within each folder, individual JavaScript files represent different algorithm implementations.
The project utilizes a centralized approach, where each algorithm is implemented as a standalone function or class. This modular design allows for easy reuse and integration into other projects. Developers can simply import the desired algorithm implementation and use it within their applications.
The project also follows established design patterns and architectural principles to ensure code maintainability and extensibility. Common design patterns such as the Singleton, Strategy, and Builder patterns are employed where appropriate. Additionally, the repository emphasizes clean code practices and adheres to industry best practices for code organization and readability.
Contribution Guidelines:
TheAlgorithms/JavaScript project encourages contributions from the open-source community, allowing developers to enhance the existing algorithms and add new ones. The project maintains specific guidelines for submitting bug reports, feature requests, and code contributions, ensuring a smooth collaboration process.
To contribute to the project, developers can follow these guidelines:
a. Fork the repository: Developers start by forking the main repository to their own GitHub account.
b. Create a new branch: Developers create a new branch for their contributions. This ensures that the main repository remains stable.
c. Make the changes: Developers make the desired changes or additions to the algorithm implementation, ensuring adherence to coding standards and practices.
d. Test the changes: Developers run the existing test suite to ensure that their changes do not introduce new bugs or regressions.
e. Submit a pull request: Developers submit a pull request from their branch to the main repository. The pull request includes a detailed description of the changes made and the problem it solves.
f. Review and merge: The project maintainers review the code changes, providing feedback and suggesting improvements if necessary. Once the code changes pass the review process, they are merged into the main repository.
The project follows a coding style guide to ensure consistency across the codebase. Additionally, developers are encouraged to provide comprehensive documentation for new algorithm implementations, including usage examples, complexity analysis, and potential edge cases.
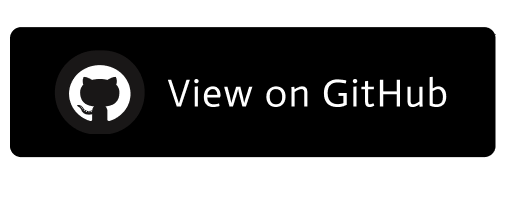